Entity Framework and Domain Driven Design Testability
I'm using a DDD approach for the Domain classes. Although, I have a problem on my design, that I'm handling it now but didn't have a good idea to over tackle it. My Architecture is the follow one: - Core Application(here I have a bunch of command/queries that use Domain entities and CQRS to process use cases) Domain -Services -Infrastructure -Presentation My problem relies in the following thing. I have a class called template that implements the interface ITemplate. This interface implements several methods and properties. As we can see above public interface ITemplate { public int Id { get; set; } string Name { get; set; } string Definition { get; set; } ISource Source { get; set; } ITemplateDefinition GetTemplateDefinitionObject(); void SetTemplateDefinition(string templateDefinitionString); } Inside a command, placed on the Application folder, I have the following: public async Task Handle(UpdateTemplateCommand request, CancellationToken cancellationToken) { var templateDto = request.Template; var sourceForTemplate = await SourceRepository.SingleAsync(x => x.Id == templateDto.SourceId); if (sourceForTemplate == null) { throw new NotFoundException(nameof(Domain.Entities.Source), request.Template.SourceId); } var templateToUpdate = await TemplateRepository.SingleAsync(x => x.Id == templateDto.Id); if (templateToUpdate == null) { throw new NotFoundException(nameof(Domain.Entities.Template), request.Template.Id); } if (!string.IsNullOrEmpty(templateDto.Definition)) { try { **templateToUpdate.SetTemplateDefinition(templateDto.Definition);** } catch (Exception e) { throw e; } } } TemplateRepository.UpdateRFEntity((Domain.Entities.Template)templateToUpdate); The line with the ** surrounding is my problem. When Unit testing this I can't fake it since the repository returns a concrete implementation and not a interface, which i think is the right way, since when dealing with a ORM like EF, with the possibility to track entities and other mechanisms we shouldn't loose that by mapping responses to a interface. Does anyone have a idea how to do it cleaner in order to be able to mock class calls without the need to make members virtual, since i have already a interface, and not having to transpose all responses to interface?
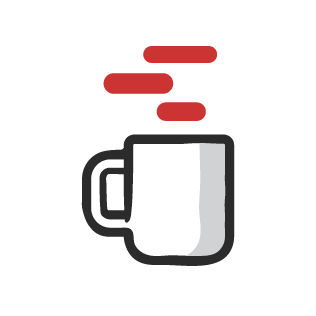
I'm using a DDD approach for the Domain classes. Although, I have a problem on my design, that I'm handling it now but didn't have a good idea to over tackle it.
My Architecture is the follow one:
- Core
- Application(here I have a bunch of command/queries that use Domain entities and CQRS to process use cases)
- Domain
-Services
-Infrastructure
-Presentation
My problem relies in the following thing. I have a class called template that implements the interface ITemplate. This interface implements several methods and properties. As we can see above
public interface ITemplate
{
public int Id { get; set; }
string Name { get; set; }
string Definition { get; set; }
ISource Source { get; set; }
ITemplateDefinition GetTemplateDefinitionObject();
void SetTemplateDefinition(string templateDefinitionString);
}
Inside a command, placed on the Application folder, I have the following:
public async Task Handle(UpdateTemplateCommand request, CancellationToken cancellationToken)
{
var templateDto = request.Template;
var sourceForTemplate = await SourceRepository.SingleAsync(x => x.Id == templateDto.SourceId);
if (sourceForTemplate == null)
{
throw new NotFoundException(nameof(Domain.Entities.Source), request.Template.SourceId);
}
var templateToUpdate = await TemplateRepository.SingleAsync(x => x.Id == templateDto.Id);
if (templateToUpdate == null)
{
throw new NotFoundException(nameof(Domain.Entities.Template), request.Template.Id);
}
if (!string.IsNullOrEmpty(templateDto.Definition))
{
try
{
**templateToUpdate.SetTemplateDefinition(templateDto.Definition);**
}
catch (Exception e)
{
throw e;
}
}
}
TemplateRepository.UpdateRFEntity((Domain.Entities.Template)templateToUpdate);
The line with the ** surrounding is my problem.
When Unit testing this I can't fake it since the repository returns a concrete implementation and not a interface, which i think is the right way, since when dealing with a ORM like EF, with the possibility to track entities and other mechanisms we shouldn't loose that by mapping responses to a interface.
Does anyone have a idea how to do it cleaner in order to be able to mock class calls without the need to make members virtual, since i have already a interface, and not having to transpose all responses to interface?